Escapia Gateway API Welcome Documentation
Overview
Getting started
The Escapia Gateway API is a Gateway API that allows Escapia partners (API users) to interact with the Escapia Vacation Rental Software (Property Management system) using JSON and the GraphQL query language. GraphQL gives clients the power to specify exactly what they need in a given request and nothing more, and makes it easier to take advantage of API evolution. The Gateway API will enable us to offer scalable and modern solutions that will improve upon or extend our existing API offerings. The first endpoint that is being made available via the Gateway API is a Rates API for Escapia inventory.
Endpoints
https://api-gateway.escapia.com/graphql
Auth token creation endpoint:
https://api-gateway.escapia.com/token
Usage
Authentication
Retrieving a bearer token:
The provided Client ID and Client Secret credentials are used to authenticate and retrieve a bearer token from the token service. A cURL request is provided below as an example token request. Replace {AUTH_TOKEN} in the request with a base64 encoded string of “ClientId:ClientSecret”.
For example, given the following client id and secret:
ClientId: 1234-555-6789
ClientSecret: 1111-111-1111
The Authorization header would be the base64 encoded string of “1234-555-6789:1111-111-1111”:
Sample cURL request for authentication (replace {AUTH_TOKEN} below with actual token):
curl -X GET \
https://api-gateway.escapia.com/token \
-H 'Authorization: Basic {AUTH_TOKEN}' \
-H 'Host: api-gateway.escapia.com' \
-H 'accept-encoding: gzip, deflate' \
-H 'cache-control: no-cache'
The body of the response will be a bearer token such as:
Requests to the GraphQL endpoint should include the bearer token as the authorization header:
Token Expiration
max-expires-date: 2020-02-03T12:19:40.097Z
Usually "Maximum expiration date" will be a constant value for the same token, still, token may expire sooner for rare cases. Because of this, it is recommended to implement a token refresh strategy. Key suggestion:
- Always retrieve new Token after max-expires-date occurs. Before this date occurs - response will contain the same token with the same expiration date;
- For rare cases: if your graphql request responded with 401 unauthorized - attempt to retrieve new token even though max-expires-date not occurred yet.
Format
curl -X POST \
https://api-gateway.escapia.com/graphql \
-H 'Accept: application/json' \
-H 'Authorization: Bearer {BEARER_TOKEN}' \
-H 'Accept-Encoding: gzip, deflate, br' \
-H 'Host: api-gateway.escapia.com' \
-H 'Content-Type: application/json' \
-d '{"query":"{ myGraphQLQuery }"}'
Rate Limiting
We've added rate limiting to help ensure the integrity of our systems and to protect our partners from unreliability caused by excessive and erratic API calls.
We reserve the right to change the limits that trigger throttling based on our system needs. Consequently we will not publish the exact limits. The rate limits will act as an upper bound that protects against API ‘attacks’. We do not anticipate these rates significantly affecting your applications. The majority of API users will not be affected by these limits, as long as they are calling the API at a reasonable rate.
If you would like to take a proactive approach, we will be returning new headers with every response:
Header | Description |
---|---|
X-RateLimit-Limit | Number of API calls that can be made during the time window |
X-RateLimit-Remaining | Remaining number of calls that can be made during the time window |
X-RateLimit-Window | Duration of time window, in milliseconds |
- X-RateLimit-Limit header of 500
- X-RateLimit-Remaining header of 499
- X-RateLimit-Window header of 60000
that would mean that the application could make up to 500 calls per minute (since the X-RateLimit-Window value is 60,000 ms = 1 minute). However, since the X-RateLimit-Remaining value is 499, only 499 more calls can be made during the window.
When your application receives a response with a “TooManyRequests” error code, you can optionally implement retry logic. Please specify a backoff. In other words, please specify an increasingly long delay between successive retries. A good starting delay value would be the same as the X-RateLimit-Window value.
Property Manager & Units
Example Queries
Property Managers enabled for integration with your application.
Request
{
myPropertyManagers(first: $first, after: $after, last: $last, before: $before) {
nodes {
name
propertyManagerId
}
pageInfo {
endCursor
hasNextPage
hasPreviousPage
startCursor
}
}
}
Response
{
"data": {
"myPropertyManagers": {
"nodes": [
{
"name": "Escapia Demo",
"propertyManagerId": 1016
}
],
"pageInfo": {
"endCursor": "eyJpbmRlZElWIlIjoxMDE2fQ==",
"hasNextPage": true,
"hasPreviousPage": false,
"startCursor": "eyJpbmRlZElWIlIjoxMDE2fQ=="
}
}
}
}
Retrieving list of units for a given Property Manager.
Pay attention that the following query is using relay pagination.
Request
{
myPropertyManagersDistributedUnitIds(propertyManagerId: $propertyManagerId, first: $first, after: $after, last: $last, before: $before) {
nodes {
unitId
}
pageInfo {
endCursor
hasNextPage
startCursor
hasPreviousPage
}
}
}
Response
{
"data": {
"myPropertyManagersDistributedUnitIds": {
"nodes": [
{
"unitId": 174962
},
{
"unitId": 174963
}
],
"pageInfo": {
"endCursor": "eyJpbmRleFZhbHVlIjoxNzQ5NjN9",
"hasNextPage": true,
"startCursor": "eyJpbmRleFZhbHVlIjoxNzQ5NjJ9",
"hasPreviousPage": false
},
}
}
}
Rates
Example Queries for Rates, Fees, and Taxes
The following queries provide example structures that can be used for retrieving rates, fees, and taxes. Given that both of these queries have the capability to retrieve the same data, the recommended approach is to use the unitsByLastRateUpdate operation.
Available Operations:
unitsByLastRateUpdate (recommended approach)
unitRates
Retrieving Recently Updated Unit Rates with:
unitsByLastRateUpdate
The following query can be used to retrieve units with recent rate updates. This query is paged and allows retrieving rates for multiple units at a time. To prevent extraneously large response payloads, the query is limited to return up to 20 records at a time. The ratesUpdatedAfter date is in ISO 8601 format, if the timezone offset is not specified UTC-0000 will be assumed.
Request
{
propertyManager(propertyManagerId: 1020) {
unitsByLastRateUpdate(ratesUpdatedAfter: “2019-01-01 13:30:00.000”, skip: 0, take: 20) {
unitId,
unitRates {
baseRent{
lengthOfStay { csv }
}
}
}
}
}
Response
{
"data": {
"propertyManager": {
"unitsByLastRateUpdate": [
{
"unitId": 39955,
"unitRates": {
"baseRent": {
"lengthOfStay": {
"csv": [
"2019-09-11,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-09-12,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-09-13,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0"
]
...
Retrieving Rates for a Single Unit with:
unitRates
The following query can be used to retrieve the length of stay rates for a singe unit.
Base Request
{
propertyManager(propertyManagerId: 1020) {
unit(unitId: 39955) {
unitId
unitRates {
baseRent {
lengthOfStay {
csv
}
}
}
}
}
}
Sample Response (Abbreviated)
{
"data": {
"propertyManager": {
"unit": {
"unitId": 39955,
"unitRates": {
"baseRent": {
"lengthOfStay": {
"csv": [
"2024-03-01,6,0,0,0,0,2775.00,0,3885.00,4440.00,4995.00,5550.00,6105.00,6660.00,0,7770.00,8325.00,8880.00,9435.00,9990.00,10545.00,0,11655.00,12210.00,12765.00,13320.00,13875.00,14430.00,0,15540.00,16095.00,16650.00,17205.00",
"2024-03-02,6,0,0,0,0,0,3330.00,3885.00,4440.00,4995.00,5550.00,6105.00,0,7215.00,7770.00,8325.00,8880.00,9435.00,9990.00,0,11100.00,11655.00,12210.00,12765.00,13320.00,13875.00,0,14985.00,15540.00,16095.00,16650.00,17205.00",
"2024-03-03,6,0,0,0,0,2775.00,3330.00,3885.00,4440.00,4995.00,5550.00,0,6660.00,7215.00,7770.00,8325.00,8880.00,9435.00,0,10545.00,11100.00,11655.00,12210.00,12765.00,13320.00,0,14430.00,14985.00,15540.00,16095.00,16650.00,17205.00",
...
Example Query: Using Optional maxStayLength Argument
"maxStayLength" accepts a value of "UpTo31Nights" or "MaxAvailable".
"UpTo31Nights" is used as the default if no argument is provided.
Length of Stay Rates is an Array of CSV strings. Each CSV string represents rates for stays up to x nights long.
"UpTo31Nights" returns Length of Stay Rates for stays up to 31 nights long.
"MaxAvailable" returns Length of Stay Rates for stays up to the maximum available. This maximum is currently set to 100 nights long.
(note: such arguments can be used with both the unitsByLastRateUpdate and discrete unitRates structures)
Request
{
propertyManager(propertyManagerId: 7007) {
unitsByLastRateUpdate(ratesUpdatedAfter: "2023-10-02 13:30:00.000", skip: 0, take: 20) {
unitId
unitRates {
baseRent {
lengthOfStay(maxStayLength: MaxAvailable) {
csv
}
}
}
}
}
}
Response
{
"data": {
"propertyManager": {
"unitsByLastRateUpdate": [
{
"unitId": 78876,
"unitRates": {
"baseRent": {
"lengthOfStay": {
"csv": [
"2023-10-02,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-03,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-04,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
...
Example Query: Using Fragments to Query for Multiple Units
Fragments can be used to include multiple queries in a single request. Below is a sample request with queries for rates for two units.
(note: fragments can be used with both the unitsByLastRateUpdate and discrete unitRates structures)
Request
fragment taxRule on TaxRule {
amount
isPercent
applyPer
name
taxRuleId
isTaxable
isSecondary
taxAuthority
stayDateRange {
min
max
}
bookDateRange {
min
max
}
stayLengthRange {
min
max
}
}
{
propertyManager(propertyManagerId: 2197) {
unit(unitId: 121792) {
unitId
unitRates {
fees {
applicableTaxRules {
...taxRule
}
}
taxRules {
...taxRule
}
}
}
}
}
Sample Response
{
"data": {
"unit": {
"unitId": 121792,
"fees":[],
"taxRules":[
{
"amount":6,
"isPercent":true,
"applyPer":"Night",
"name":"3000.1000",
"taxRuleId":"7216",
...
Example Query: Revealing Hidden Fields
"fees": [
{
"name": "IN_RENT_FEE",
"description": "IN_RENT_FEE",
"amount": 5,
"includeInRent": true,
"feeId": 3213,
"productCode": "MANAGEMENT"
},
{
"name": "Miscellaneous Booking Charge",
"description": "Parking Pass @ $15/week",
"amount": 15,
"includeInRent": false,
"feeId": 1231,
"productCode": "PARKING"
}
]
Such fields can be revealed by using argument showActualValue: true
(note: such arguments can be used with both the unitsByLastRateUpdate and discrete unitRates structures)
Request
{
propertyManager(propertyManagerId: 7007) {
unitsByLastRateUpdate(ratesUpdatedAfter: "2023-10-02 13:30:00.000", skip: 0, take: 20) {
unitId
unitRates {
fees {
name(showActualValue: true)
description(showActualValue: true)
amount
includeInRent
feeId
}
}
}
}
}
Sample Response (Abbreviated)
{
"data": {
"propertyManager": {
"unitsByLastRateUpdate": [
{
"unitId": 78876,
"unitRates": {
"fees": [
{
"name": "Miscellaneous Booking Charge",
"description": "Group With Rent Per Night Charge",
"amount": 20,
"includeInRent": true,
"feeId": 23390
},
{
"name": "Booking Pet Charge",
"description": "Pet Charges",
"amount": 50,
"includeInRent": false,
"feeId": 39971
},
]
}
}
]
}
}
}
Pricing Engine
Retrieve active pricing engine for a property manager
- Rules based Rates Editor (old version)
- Rates Manager (new version)
The Nightly Rates and Booking Restrictions outlined below are only supported by Rates Manager.
These nightly rates and booking restrictions will not be used to generate prices, including Length of Stay Rates, for travelers until the Property Manager has chosen to switch the System of Record for pricing from the old to the new engine.
The Pricing Engine cannot be changed through this API.
Engine | Description |
---|---|
RATES_MANAGER | New Rates Manager pricing engine. Nightly Rates and Booking Restrictions will be used to calculate LoS Rates. |
OTHER | Old pricing engine |
Sample GraphQL Schema
enum PricingEngine {
RATES_MANAGER
OTHER
}
type PropertyManager {
pricingEngine: PricingEngine
}
- Pricing Engine can only be queried for a single property manager at a time
Sample Request
query {
myPartnerAccount {
propertyManager(propertyManagerId: 1234) {
pricingEngine
}
}
}
Sample Response
{
"data": {
"myPartnerAccount": {
"propertyManager": {
"pricingEngine": "RATES_MANAGER"
}
}
}
}
Nightly Rates
Retrieve nightly rates for a unit
The "date" is the night of that date e.g. "2020-01-01" is the night of "2020-01-01" -> "2020-01-02"
Constraints:
- Nightly Rates can only be queried for a single property manager at a time
- Nightly Rates can only be queried for a single unit at a time
- The provided date range is inclusively constrained by the date range today -> 2 years from today
- The provided date range cannot be null
- The provided start and end dates cannot be null
Sample Request
query {
myPartnerAccount {
propertyManager(propertyManagerId: 1234) {
unit(unitId: 654321) {
rates {
nightlyRatesByDateRange(filter: {
dateRange: {start: "2020-01-01" end: "2020-01-03"}
}) {
nightlyRate
date
}
}
}
}
}
}
Sample Response
{
"data": {
"myPartnerAccount": {
"propertyManager": {
"unit": {
"rates": {
"nightlyRatesByDateRange": [
{
"nightlyRate": 100,
"date": "2020-01-01"
},
{
"nightlyRate": 101,
"date": "2020-01-02"
},
{
"nightlyRate": 102,
"date": "2020-01-03"
}
]
}
}
}
}
}
}
Set nightly rates for a unit
Sample GraphQL Schema
input NightlyRateInput {
date: Date!
rate: Int!
}
input Rate_SetUnitNightlyRatesByDatesInput {
propertyManagerId: Int!
unitId: Int!
nightlyRates: [NightlyRateInput]!
}
type Rate_SetUnitNightlyRatesByDatesPayload {
success: Boolean!
}
type Mutation {
rate_SetUnitNightlyRatesByDates(
input: Rate_SetUnitNightlyRatesByDatesInput!
): Rate_SetUnitNightlyRatesByDatesPayload!
}
- The provided dates are inclusively constrained by the date range today -> 2 years from today
- The provided dates cannot be null
- The provided rates are inclusively constrained by a minimum of 1 and a maximum of 50000
- The provided rates must be Integers
- The provided rates cannot be null
- Nightly Rates cannot be removed using this mutation
- If any date and rate pair is invalid, the whole request will fail
Sample Request
mutation {
rate_SetUnitNightlyRatesByDates(input: {
propertyManagerId: 1234,
unitId: 654321,
nightlyRates: [
{date: "2020-02-01", rate: 100},
{date: "2020-01-02", rate: 101},
{date: "2020-01-01", rate: 102},
]
}) {
success
}
}
Sample Response
{
"data": {
"rate_SetUnitNightlyRatesByDates": {
"success": true
}
}
}
Booking Restrictions
Retrieve booking restrictions for a unit
For a given date:
- checkInAllowed is a Boolean representing a traveler can check in on that date
- checkoutAllowed is a Boolean representing a traveler can check out on that date
- minimumNightStay is an Integer representing the minimum number of days a traveler must stay for a stay including that date
dateLevelOverride VS effectiveValue:
The dateLevelOverride is the value set at that specific date. The effectiveValue overrides the global value with the date value (if one exists).
global value | date value | dateLevelOverride | effectiveValue |
---|---|---|---|
null | null | null | null |
null | populatedDateValue | populatedDateValue | populatedDateValue |
populatedGlobalValue | null | null | populatedGlobalValue |
populatedGlobalValue | populatedDateValue | populatedDateValue | populatedDateValue |
Constraints:
- Booking Restrictions can only be queried for a single property manager at a time
- Booking Restrictions should only be queried for a single unit at a time
- The provided date range is inclusively constrained by the date range today -> 2 years from today
- The provided date range cannot be null
- The provided start and end dates cannot be null
Sample Request
query {
myPartnerAccount {
propertyManager(propertyManagerId: 1234) {
unit(unitId: 654321) {
bookingRestrictions {
bookingRestrictionsByDateRange(filter: {
dateRange: {start: "2020-01-01" end: "2020-01-02"}
}) {
date
checkInAllowed {
dateLevelOverride
effectiveValue
}
checkoutAllowed {
dateLevelOverride
effectiveValue
}
minimumNightStay {
dateLevelOverride
effectiveValue
}
}
}
}
}
}
}
Sample Response
{
"data": {
"myPartnerAccount": {
"propertyManager": {
"unit": {
"bookingRestrictions": {
"bookingRestrictionsByDateRange": [
{
"date": "2020-01-01",
"checkInAllowed": {
"dateLevelOverride": true,
"effectiveValue": true
},
"checkoutAllowed": {
"dateLevelOverride": false,
"effectiveValue": false
},
"minimumNightStay": {
"dateLevelOverride": 1,
"effectiveValue": 1
}
},
{
"date": "2020-01-02",
"checkInAllowed": {
"dateLevelOverride": null,
"effectiveValue": false
},
"checkoutAllowed": {
"dateLevelOverride": null,
"effectiveValue": true
},
"minimumNightStay": {
"dateLevelOverride": null,
"effectiveValue": 2
}
}
]
}
}
}
}
}
}
Set date booking restrictions for a unit
Sample GraphQL Schema
input DateBookingRestrictionsInput {
date: Date!
minimumNightStay: Int
isCheckInAllowed: Boolean
isCheckoutAllowed: Boolean
}
input BookingRestriction_SetDateBookingRestrictionsByUnitInput {
propertyManagerId: Int!
unitId: Int!
dateBookingRestrictions: [DateBookingRestrictionsInput!]!
}
type BookingRestriction_SetDateBookingRestrictionsByUnitPayload{
success: Boolean!
}
type Mutation {
bookingRestriction_SetDateBookingRestrictionsByUnit(
input: BookingRestriction_SetDateBookingRestrictionsByUnitInput!
): BookingRestriction_SetDateBookingRestrictionsByUnitPayload!
}
- The provided dates are inclusively constrained by the date range today -> 2 years from today
- The provided dates cannot be null
- The provided minimumNightStays are inclusively constrained by a minimum of 1 and a maximum of 98
- The provided minimumNightStays must be Integers
- The provided isCheckInAlloweds must be Booleans
- The provided isCheckoutAlloweds must be Booleans
- Date Booking Restrictions cannot be removed using this mutation
- If any date booking restriction is invalid, the whole request will fail
Sample Request
mutation {
bookingRestriction_SetDateBookingRestrictionsByUnit(input: {
propertyManagerId: 1234,
unitId: 654321,
dateBookingRestrictions: [
{date: "2020-02-01", minimumNightStay: 4, isCheckInAllowed: true, isCheckoutAllowed: false},
{date: "2020-01-02", minimumNightStay: 5, isCheckInAllowed: false, isCheckoutAllowed: false},
{date: "2020-01-01", minimumNightStay: 6, isCheckInAllowed: true, isCheckoutAllowed: true},
]
}) {
success
}
}
Sample Response
{
"data": {
"bookingRestriction_SetDateBookingRestrictionsByUnit": {
"success": true
}
}
}
Enum values
applyPer
Stay
Night
Week
Month
productCode
CLEANING
GUEST
PET
ADMINISTRATIVE
MANAGEMENT
RESORT
POOL
PARKING
HOT_TUB
LINENS
BOOKING
RESERVATION
WAIVER_DAMAGE
LABOR
CONCIERGE
Errors
Unit Rate not found
For certain units it is possible to receive error like this:
Sample error
{
"errors": [
{
"message": "Unit rate not found",
"path": [
"_entities",
0,
"unitRates"
],
}
]
}
This indicates that unit may not have properly configured rates. To solve this please configure rates in Escapia UI or contact Property Manager.
Booking Channels
Retrieve all known Booking Channels
This method provides available booking channels that can be used in create Reservation request.
Sample Request
query {
bookingChannels {
value
displayName
}
}
Sample Response
{
"data": {
"bookingChannels": [
{
"value": "ABRITEL",
"displayName": "Abritel",
},
{
"value": "ADRIATIC_HOME",
"displayName": "Adriatic Home",
},
{
"value": "AEGEAN_AIRLINES",
"displayName": "Aegean Airlines",
},
...
]
}
}
Appendix
Recommended call frequency
- Distributed Property Manager Names and IDs - Call once every 2 hours.
- Distributed Units - Call once every 2 hours.
- If a new unit is returned, it should trigger an ad hoc call for the full listing content query for that unit.
- If only using the GraphQL listing content endpoint for bedroom/bathroom configurations, it should trigger an ad hoc call of the Bedroom and Bathroom query.
- Full Listing Content Query - Call once per day.
- LastUpdated Listing Content Query - Call once every 4 hours.
- If any units are returned in this call, it should trigger an ad hoc call of the full listing content query for those units.
- Bedroom and Bathroom Query - Call once per day.
- Only use this query if you're using the GraphQL listing content endpoint for bedroom/bathroom configurations.
- Do not use this query if using the full listing content query, as bedrooms and bathrooms are included in that query.
- getUnitRates - Call once per day.
- unitsByLastRateUpdate - Call once every 4 hours.
- NightlyRatesByDateRange - Call once every 4 hours.
- unitRates(Fees and Taxes) - Call once every 4 hours.
- unitRates → Base Rent - Call once every 4 hours.
- NightlyRatesByDateRange - Call once every 4 hours.
- BookingRestrictionsByDateRange - Call once every 4 hours.
Deprecated queries
getDistributedUnits
- Use "myPartnerAccount" { myProperties { ... }}
getAuthorizedPropertyManagers
- Use "myPartnerAccount" { myPropertyManagers { ... }}
myPartnerAccount.myProperties
- Use "myPartnerAccount" { propertyManager(...) { distributedUnits(...) { ... }}}
myPartnerAccount.myPropertyManagers.units
- Use "myPartnerAccount" { myPropertyManagers { distributedUnits(...) { ... }}}
Other API Usage Considerations
Accept-Encoding: gzip, deflate, br
Fees and taxRules
- We support various systems and are sensitive to each system requirements. Some of these systems use per "night" on percentage based taxes.
- However, A percentage amount applied per "night" or per "stay" is exactly the same, therefore "applyPer = night" can be ignored for percentage based fees and taxes.
Sample Response Payload (unit fees and taxRules)
{
"data": {
"myPartnerAccount": {
"unit121792": {
... removed for brevity,
"fees": [
{
"name": "Booking Fee",
"description": "Reservation Fee",
"applyPer": "Stay",
"amount": 5,
"includeInRent": true,
"feeId": 3213
},
{
"name": "Miscellaneous Booking Charge",
"description": "Parking Pass @ $15/week",
"applyPer": "Stay",
"amount": 15,
"includeInRent": false,
"feeId": 1231
}
],
"taxRules": [
{
"amount": 6,
"isPercent": true,
"applyPer": "Night",
"name": "3000.1000",
"taxRuleId": "7216",
"isTaxable": false,
"isSecondary": false,
"taxAuthority": "Dare - Occupancy TAX",
"stayDateRange": {
"min": "2017-01-01T00:00:00Z",
"max": "2017-12-31T00:00:00Z"
},
"bookDateRange": null,
"stayLengthRange": {
"min": 1,
"max": 90
}
},
{
"amount": 6.75,
"isPercent": true,
"applyPer": "Night",
"name": "3000.1010",
"taxRuleId": "7217",
"isTaxable": false,
"isSecondary": false,
"taxAuthority": "Dare - NC St TAX",
"stayDateRange": {
"min": "2017-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"bookDateRange": null,
"stayLengthRange": {
"min": 1,
"max": 90
}
}
]
}
}
}
}
"includeInRent" Fees
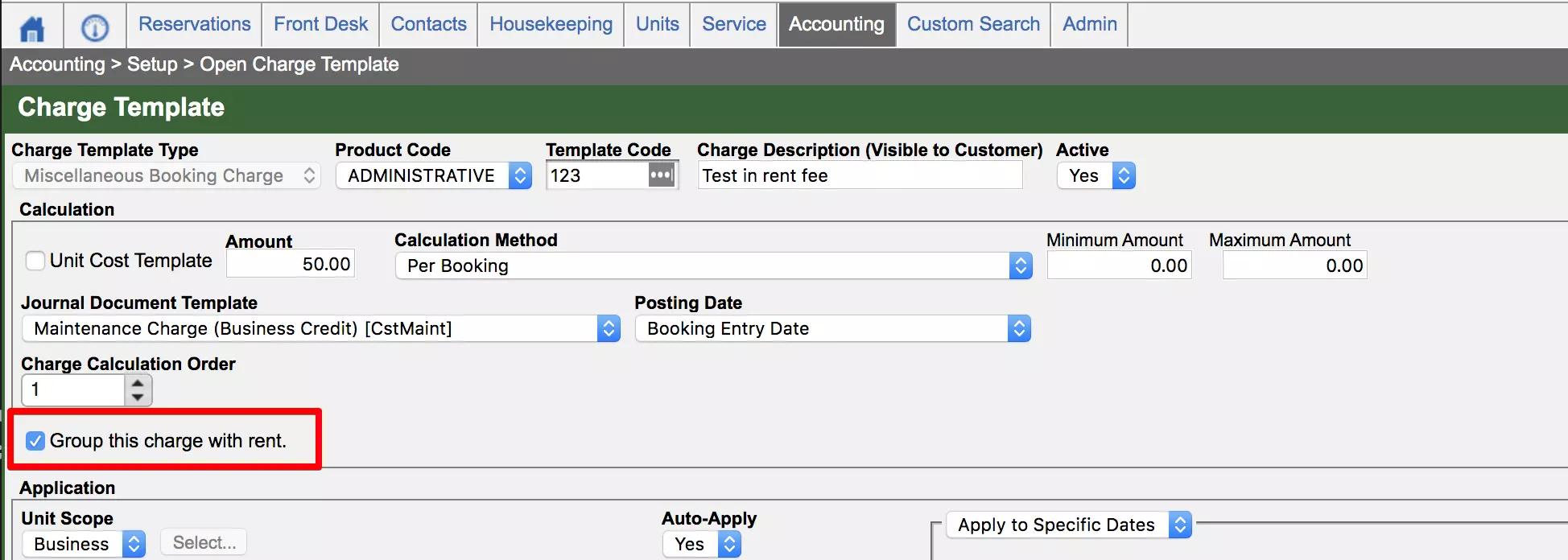
These fees are not included in the LOS baseRent, but when quoting they should be rolled into the baseRent and should not be displayed to a traveler as a separate line item. See the example below:
With out in-rent fee: | With booking charge as in-rent: |
---|---|
Rent: $100 | Rent: $125 |
Fees: * Booking Charge: $25 Housekeeping: $50 | Fees: Housekeeping: $50 |
Sample “includeInRent” Fee
{
"fees": [
{
"name": "Miscellaneous Booking Charge",
"description": "Test in rent fee",
"isPercent": false,
"amount": 50,
"applyPer": "Stay",
"includeInRent": true,
"productCode": "ADMINISTRATIVE",
"feeId": 75791
}
]
}
"includeInRent" Fees Detailed Example
Sample Request
{
getUnitRates(propertyManagerId: 1020, unitId: 40067)
{
unitId
baseRent {
lengthOfStay { csv}
},
fees {
name(showActualValue: true),
description(showActualValue: true),
isPercent,
amount,
applyPer,
includeInRent,
productCode,
feeId,
}
}
}
Sample Response (Abbreviated. Up to 365 days of rates may be included.)
{
"data": {
"getUnitRates": {
"unitId": "21506",
"baseRent": {
"lengthOfStay": {
"csv": [
"2019-12-16,4,340.00,680.00,1020.00,1200.00,1500.00,1800.00,1500.00,1800.00,2100.00,2400.00,2700.00,3000.00,3300.00,3000.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-17,4,340.00,680.00,1020.00,1360.00,1700.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-18,4,340.00,680.00,1020.00,1360.00,1700.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-19,4,340.00,680.00,1020.00,1360.00,1700.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0"
]
}
},
"fees": [
{
"name": "Booking Pet Charge",
"description": "Pet Fee",
"isPercent": false,
"amount": 25,
"applyPer": "Stay",
"includeInRent": false,
"productCode": "PET",
"feeId": 71224
},
{
"name": "Miscellaneous Booking Charge",
"description": "Test Fee 1",
"isPercent": false,
"amount": 100,
"applyPer": "Stay",
"includeInRent": false,
"productCode": "ADMINISTRATIVE",
"feeId": 71787
},
{
"name": "Miscellaneous Booking Charge",
"description": "Test Fee 2",
"isPercent": false,
"amount": 200,
"applyPer": "Stay",
"includeInRent": false,
"productCode": "ADMINISTRATIVE",
"feeId": 71788
},
{
"name": "Miscellaneous Booking Charge",
"description": "Test in rent fee",
"isPercent": false,
"amount": 50,
"applyPer": "Stay",
"includeInRent": true,
"productCode": "ADMINISTRATIVE",
"feeId": 75791
},
{
"name": "Miscellaneous Booking Charge",
"description": "Admin Fee",
"isPercent": false,
"amount": 11,
"applyPer": "Stay",
"includeInRent": false,
"productCode": "BOOKING",
"feeId": 78795
}
]
}
}
}
Percentage-based fees
Our API exposes 2 types of percentage-based fees:
- Percent of Rent
- Percent of Booking Total w/o Security Deposits and/or Taxes
The first type uses just rent, whereas the second type uses rent and all other fees except for refundable damage deposits.
After introducing the new percentageOf
field under Fee
type, we are providing guidance on how to calculate the percentage-based fees.
Given Rent = $1000, Cleaning fee 1 = $50, Cleaning fee 2 = $10, Pet fee = $100
percentageOf: ["RENT"]`, amount: 10%
=> 10% of $1000 = $100
percentageOf: ["RENT", "CLEANING"], amount: 10%
=> 10% of ($1000 + $50 + $10) = $106
percentageOf: ["RENT", "CLEANING", "PET"], amount: 10%
=> 10% of ($1000 + $50 + $10 + $100) = $116
Sample Request
{
getUnitRates(propertyManagerId: 1000, unitId: 20000)
{
fees{
name,
description,
isPercent,
amount,
applyPer,
productCode,
percentageOf
}
}
}
Sample Response
{
"data": {
"getUnitRates": {
"fees": [
{
"name": "Miscellaneous Booking Charge",
"description": "Property Damage Protection",
"isPercent": false,
"amount": 99,
"applyPer": "Stay",
"productCode": "WAIVER_DAMAGE",
"percentageOf": []
},
{
"name": "Booking Fee",
"description": "Agency Services",
"isPercent": true,
"amount": 1,
"applyPer": "Stay",
"productCode": "ADMINISTRATIVE",
"percentageOf": [
"WAIVER_DAMAGE",
"CLEANING",
"RENT"
]
}
]
}
}
}
"LoS" (lengthOfStay) Unit Rates Explanation
{check-in date},{occupancy},{1-night price},{2-nights price},{3-nights price},...
- {check-in date} — Is in the format: yyyy-mm-dd. Can have up to 10 sets of prices (rows) for a specific check-in date with different occupancies.
- {occupancy} — Maximum number of guests (1-99) for the specified prices. For example, a 4 in the {occupancy} field indicates 1-4 guests can be quoted.
- {#-night price} — Each field provides the total rental rate (0-99999999.99) for the specified number of nights, excluding taxes and fees. 0 in a price field indicates that the rate is not quotable for that length of stay. There will always be 31 price fields (no more or less), and all rows will have the same number of price fields.
{
"lengthOfStay": {
"csv": [
"2019-12-16,4,340.00,680.00,1020.00,1200.00,1500.00,1800.00,1500.00,1800.00,2100.00,2400.00,2700.00,3000.00,3300.00,3000.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-17,4,340.00,680.00,1020.00,1360.00,1700.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-18,4,340.00,680.00,1020.00,1360.00,1700.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-19,4,340.00,680.00,1020.00,1360.00,1700.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0"
]
}
}
Relay Pagination Explanation
Here is the example request with relay pagination and it's response:
Request
{
myPropertyManagersDistributedUnitIds(propertyManagerId: $propertyManagerId, first: $first, after: $after, last: $last, before: $before) {
totalEdges
pageInfo {
endCursor
hasNextPage
startCursor
hasPreviousPage
}
nodes {
unitId
}
edges {
cursor
node {
unitId
}
}
}
}
Response
{
"data": {
"myPropertyManagersDistributedUnitIds": {
"totalEdges": 3,
"pageInfo": {
"endCursor": "eyJpbmRleFZhbHVlIjoyMzgzMTB9",
"hasNextPage": false,
"startCursor": "eyJpbmRleFZhbHVlIjoyMTcwNDV9",
"hasPreviousPage": false
},
"nodes": [
{
"unitId": 217045
},
{
"unitId": 217095
},
{
"unitId": 238310
}
],
"edges": [
{
"cursor": "eyJpbmRleFZhbHVlIjoyMTcwNDV9",
"node": {
"unitId": 217045
}
},
{
"cursor": "eyJpbmRleFZhbHVlIjoyMTcwOTV9",
"node": {
"unitId": 217095
}
},
{
"cursor": "eyJpbmRleFZhbHVlIjoyMzgzMTB9",
"node": {
"unitId": 238310
}
}
]
}
}
}
Relay pagination params:
- first — list first items amount to take
- last — list last items amount to take
- before — item ID to take items before
- after — item ID to take items after
Can be used next relay pagination params combinations:
- (first: "some number", after: "some ID")
- (last: "some number", before: "some ID")
- each param separately
- none of params (default params values will be used)
Relay pagination response:
- edges - items (nodes) and their cursors (IDs) list
- nodes - items list
- pageInfo - pagination information:
- startCursor - first item ID
- endCursor - last item ID
- hasNextPage - boolean value which indicates does there next pages (items)
- hasPreviousPage - boolean value which indicates does there previous pages (items)
Additional resources
Full LOS Rates Response Sample
{
"data": {
"myPartnerAccount": {
"propertyManager": {
"unit121792": {
"unitId": 121792,
"unitRates": {
"configuration": {
"currency": "USD"
},
"baseRent": {
"lengthOfStay": {
"csv": [
"2019-09-15,6,0,0,0,0,0,0,1120.00,0,0,0,0,0,0,2201.00,0,0,0,0,0,0,3282.00,0,0,0,0,0,0,0,0,0,0",
"2019-09-22,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,2162.00,0,0,0,0,0,0,3242.00,0,0,0,0,0,0,0,0,0,0",
"2019-09-29,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,2162.00,0,0,0,0,0,0,3242.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-06,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,2162.00,0,0,0,0,0,0,3001.59,0,0,0,0,0,0,0,0,0,0",
"2019-10-13,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,1920.58,0,0,0,0,0,0,2760.58,0,0,0,0,0,0,0,0,0,0",
"2019-10-20,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-21,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-22,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-23,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-24,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-25,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-26,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-27,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-28,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-29,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-30,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-31,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-01,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-02,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-03,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-04,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-05,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-06,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-07,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-08,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-09,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-10,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-11,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-12,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-13,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-14,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-15,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-16,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-17,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-18,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-19,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-20,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-21,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-22,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1195.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-23,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1075.00,1190.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-24,6,0,0,480.00,640.00,800.00,960.00,840.00,955.00,1070.00,1185.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-25,6,0,0,480.00,640.00,800.00,960.00,835.00,950.00,1065.00,1180.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-26,6,0,0,480.00,640.00,800.00,915.00,830.00,945.00,1060.00,1175.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-27,6,0,0,480.00,640.00,755.00,870.00,825.00,940.00,1055.00,1170.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-28,6,0,0,480.00,595.00,710.00,825.00,820.00,935.00,1050.00,1165.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-29,6,0,0,435.00,550.00,665.00,780.00,815.00,930.00,1045.00,1160.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-30,6,0,0,390.00,505.00,620.00,735.00,810.00,925.00,1040.00,1155.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-01,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-02,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-03,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-04,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-05,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-06,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-07,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-08,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-09,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-10,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-11,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-12,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-13,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,950.64,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-14,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,858.68,982.16,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-15,6,0,0,345.00,460.00,575.00,690.00,805.00,766.65,890.14,1012.86,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-16,6,0,0,345.00,460.00,575.00,690.00,674.64,798.12,920.88,1044.24,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-17,6,0,0,345.00,460.00,575.00,698.00,706.15,828.90,952.20,1075.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-18,6,0,0,345.00,460.00,583.00,706.00,736.88,860.20,983.00,1105.84,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-19,6,0,0,345.00,468.00,591.00,714.00,768.15,891.00,1013.88,1137.06,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-20,6,0,0,353.00,476.00,599.00,722.00,799.00,921.90,1045.04,1167.96,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-21,6,0,0,361.00,484.00,607.00,730.00,829.95,953.02,1075.98,1199.04,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-22,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,1181.00,1279.00,1378.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-23,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,1181.00,1279.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-24,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,1181.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-25,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-26,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,984.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-27,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,886.00,801.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-28,6,0,0,369.00,492.00,615.00,738.00,861.00,787.00,722.00,814.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-29,6,0,0,369.00,492.00,615.00,738.00,689.00,643.00,735.00,827.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-30,6,0,0,369.00,492.00,615.00,590.00,564.00,656.00,748.00,840.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-31,6,0,0,369.00,492.00,492.00,486.00,578.00,670.00,762.00,854.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-01,6,0,0,369.00,492.00,407.00,499.00,591.00,683.00,775.00,867.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-02,6,0,0,369.00,484.00,420.00,512.00,604.00,696.00,788.00,880.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-03,6,0,0,361.00,476.00,434.00,525.00,617.00,709.00,801.00,893.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-04,6,0,0,353.00,468.00,447.00,539.00,631.00,723.00,815.00,907.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-05,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-06,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-07,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-08,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-09,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-10,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-11,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-12,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-13,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-14,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-15,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-16,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-17,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-18,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-19,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-20,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-21,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-22,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-23,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-24,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-25,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-26,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-27,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-28,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-29,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-30,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-31,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-01,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-02,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-03,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-04,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-05,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-06,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-07,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-08,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-09,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-10,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-11,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-12,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-13,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-14,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-15,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-16,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-17,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-18,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-19,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-20,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-21,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-22,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-23,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-24,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-25,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-26,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-27,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-28,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-29,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-01,6,0,0,345.00,460.00,460.00,552.00,644.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-02,6,0,0,345.00,460.00,460.00,552.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-03,6,0,0,345.00,460.00,460.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-04,6,0,0,345.00,460.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-05,6,0,0,345.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0"
]
}
},
"fees": [],
"taxRules": [
{
"amount": 6,
"isPercent": true,
"applyPer": "Night",
"name": "3000.1000",
"taxRuleId": "7216",
"isTaxable": false,
"isSecondary": false,
"taxAuthority": "Dare - Occupancy TAX",
"stayDateRange": {
"min": "2017-01-01T00:00:00Z",
"max": "2017-12-31T00:00:00Z"
},
"bookDateRange": null,
"stayLengthRange": {
"min": 1,
"max": 90
}
},
{
"amount": 6.75,
"isPercent": true,
"applyPer": "Night",
"name": "3000.1010",
"taxRuleId": "7217",
"isTaxable": false,
"isSecondary": false,
"taxAuthority": "Dare - NC St TAX",
"stayDateRange": {
"min": "2017-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"bookDateRange": null,
"stayLengthRange": {
"min": 1,
"max": 90
}
}
]
}
},
"unit121793": {
"unitId": 121793,
"unitRates": {
"configuration": {
"currency": "USD"
},
"baseRent": {
"lengthOfStay": {
"csv": [
"2019-09-15,6,0,0,0,0,0,0,1120.00,0,0,0,0,0,0,2201.00,0,0,0,0,0,0,3282.00,0,0,0,0,0,0,0,0,0,0",
"2019-09-22,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,2162.00,0,0,0,0,0,0,3242.00,0,0,0,0,0,0,0,0,0,0",
"2019-09-29,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,2162.00,0,0,0,0,0,0,3242.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-06,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,2162.00,0,0,0,0,0,0,3001.59,0,0,0,0,0,0,0,0,0,0",
"2019-10-13,6,0,0,0,0,0,0,1081.00,0,0,0,0,0,0,1920.58,0,0,0,0,0,0,2760.58,0,0,0,0,0,0,0,0,0,0",
"2019-10-20,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-21,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-22,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-23,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-24,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-25,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-26,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-27,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-28,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-29,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-30,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-10-31,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-01,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-02,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-03,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-04,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-05,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-06,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-07,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-08,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-09,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-10,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,2520.00,0,0,0,0,0,0,0,0,0,0",
"2019-11-11,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,2400.00,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-12,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,2280.00,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-13,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,2160.00,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-14,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,2040.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-15,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,1920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-16,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,1800.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-17,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,1680.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-18,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,1560.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-19,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,1440.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-20,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,1320.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-21,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1200.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-22,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1080.00,1195.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-23,6,0,0,480.00,640.00,800.00,960.00,840.00,960.00,1075.00,1190.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-24,6,0,0,480.00,640.00,800.00,960.00,840.00,955.00,1070.00,1185.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-25,6,0,0,480.00,640.00,800.00,960.00,835.00,950.00,1065.00,1180.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-26,6,0,0,480.00,640.00,800.00,915.00,830.00,945.00,1060.00,1175.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-27,6,0,0,480.00,640.00,755.00,870.00,825.00,940.00,1055.00,1170.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-28,6,0,0,480.00,595.00,710.00,825.00,820.00,935.00,1050.00,1165.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-29,6,0,0,435.00,550.00,665.00,780.00,815.00,930.00,1045.00,1160.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-11-30,6,0,0,390.00,505.00,620.00,735.00,810.00,925.00,1040.00,1155.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-01,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-02,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-03,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-04,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-05,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-06,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-07,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-08,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-09,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-10,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-11,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-12,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,1150.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-13,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,1035.00,950.64,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-14,6,0,0,345.00,460.00,575.00,690.00,805.00,920.00,858.68,982.16,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-15,6,0,0,345.00,460.00,575.00,690.00,805.00,766.65,890.14,1012.86,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-16,6,0,0,345.00,460.00,575.00,690.00,674.64,798.12,920.88,1044.24,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-17,6,0,0,345.00,460.00,575.00,698.00,706.15,828.90,952.20,1075.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-18,6,0,0,345.00,460.00,583.00,706.00,736.88,860.20,983.00,1105.84,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-19,6,0,0,345.00,468.00,591.00,714.00,768.15,891.00,1013.88,1137.06,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-20,6,0,0,353.00,476.00,599.00,722.00,799.00,921.90,1045.04,1167.96,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-21,6,0,0,361.00,484.00,607.00,730.00,829.95,953.02,1075.98,1199.04,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-22,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,1181.00,1279.00,1378.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-23,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,1181.00,1279.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-24,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,1181.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-25,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,1230.00,1082.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-26,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,1107.00,984.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-27,6,0,0,369.00,492.00,615.00,738.00,861.00,984.00,886.00,801.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-28,6,0,0,369.00,492.00,615.00,738.00,861.00,787.00,722.00,814.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-29,6,0,0,369.00,492.00,615.00,738.00,689.00,643.00,735.00,827.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-30,6,0,0,369.00,492.00,615.00,590.00,564.00,656.00,748.00,840.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-12-31,6,0,0,369.00,492.00,492.00,486.00,578.00,670.00,762.00,854.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-01,6,0,0,369.00,492.00,407.00,499.00,591.00,683.00,775.00,867.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-02,6,0,0,369.00,484.00,420.00,512.00,604.00,696.00,788.00,880.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-03,6,0,0,361.00,476.00,434.00,525.00,617.00,709.00,801.00,893.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-04,6,0,0,353.00,468.00,447.00,539.00,631.00,723.00,815.00,907.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-05,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-06,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-07,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-08,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-09,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-10,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-11,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-12,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-13,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-14,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-15,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-16,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-17,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-18,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-19,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-20,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-21,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-22,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-23,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-24,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-25,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-26,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-27,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-28,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-29,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-30,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-01-31,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-01,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-02,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-03,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-04,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-05,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-06,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-07,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-08,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-09,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-10,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-11,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-12,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-13,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-14,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-15,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-16,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-17,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-18,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-19,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-20,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-21,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-22,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-23,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-24,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-25,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-26,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-27,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,920.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-28,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,828.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-02-29,6,0,0,345.00,460.00,460.00,552.00,644.00,736.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-01,6,0,0,345.00,460.00,460.00,552.00,644.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-02,6,0,0,345.00,460.00,460.00,552.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-03,6,0,0,345.00,460.00,460.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-04,6,0,0,345.00,460.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2020-03-05,6,0,0,345.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0"
]
}
},
"fees": [],
"taxRules": [
{
"amount": 6,
"isPercent": true,
"applyPer": "Night",
"name": "3000.1000",
"taxRuleId": "7216",
"isTaxable": false,
"isSecondary": false,
"taxAuthority": "Dare - Occupancy TAX",
"stayDateRange": {
"min": "2017-01-01T00:00:00Z",
"max": "2017-12-31T00:00:00Z"
},
"bookDateRange": null,
"stayLengthRange": {
"min": 1,
"max": 90
}
},
{
"amount": 6.75,
"isPercent": true,
"applyPer": "Night",
"name": "3000.1010",
"taxRuleId": "7217",
"isTaxable": false,
"isSecondary": false,
"taxAuthority": "Dare - NC St TAX",
"stayDateRange": {
"min": "2017-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"bookDateRange": null,
"stayLengthRange": {
"min": 1,
"max": 90
}
}
]
}
}
}
}
}
}
Full Example of fees and taxes for a unit
Request
query {
propertyManager(propertyManagerId: 7007) {
unitsByLastRateUpdate(ratesUpdatedAfter: "2023-08-01 13:30:00.000", skip: 0, take: 20) {
unitId
unitRates {
fees {
feeId
name(showActualValue: true)
description(showActualValue: true)
includeInRent
productCode
amount
applyPer
isPercent
percentageOf
stayDateRange {
min
max
}
stayLengthRange {
min
max
}
bookDateRange {
min
max
}
applicableTaxRules {
taxRuleId
name
isSecondary
taxAuthority
amount
isPercent
applyPer
stayDateRange {
min
max
}
stayLengthRange {
min
max
}
bookDateRange {
min
max
}
}
}
taxRules {
taxRuleId
name
isSecondary
taxAuthority
amount
isPercent
applyPer
stayDateRange {
min
max
}
stayLengthRange {
min
max
}
bookDateRange {
min
max
}
}
}
}
}
}
Sample Response
{
"data": {
"propertyManager": {
"unitsByLastRateUpdate": [
{
"unitId": 78876,
"unitRates": {
"fees": [
{
"feeId": 23390,
"name": "Miscellaneous Booking Charge",
"description": "Group With Rent Per Night Charge",
"includeInRent": true,
"productCode": "BOOKING",
"amount": 20,
"applyPer": "Night",
"isPercent": false,
"percentageOf": [],
"stayDateRange": {
"min": "2013-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"stayLengthRange": null,
"bookDateRange": {
"min": "0001-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"applicableTaxRules": null
},
{
"feeId": 39971,
"name": "Booking Pet Charge",
"description": "Pet Charges",
"includeInRent": false,
"productCode": "PET",
"amount": 50,
"applyPer": "Stay",
"isPercent": false,
"percentageOf": [],
"stayDateRange": {
"min": "2015-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"stayLengthRange": null,
"bookDateRange": {
"min": "0001-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"applicableTaxRules": null
},
],
"taxRules": [
{
"taxRuleId": "3288",
"name": "3000.1000",
"isSecondary": false,
"taxAuthority": "KC City Tax",
"amount": 9,
"isPercent": true,
"applyPer": "Night",
"stayDateRange": {
"min": "2006-01-01T00:00:00Z",
"max": "9999-12-31T00:00:00Z"
},
"stayLengthRange": {
"min": 1,
"max": 998
},
"bookDateRange": null
}
]
}
}
]
}
}
}
Other Full Response Samples
Full Response Sample of Retrieving Recently Updated Unit Rates with unitsByLastRateUpdate
(Abbreviated, full response includes up to 734* days of rates)
Not all agencies will define rates for 734 days, so the actual number of days returned may vary depending on agency setup.
{
"data": {
"propertyManager": {
"unitsByLastRateUpdate": [
{
"unitId": 39955,
"unitRates": {
"baseRent": {
"lengthOfStay": {
"csv": [
"2019-09-11,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-09-12,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-09-13,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0"
]
}
}
}
}, {
"unitId": 39956,
"unitRates": {
"baseRent": {
"lengthOfStay": {
"csv": [
"2019-09-11,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-09-12,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0",
"2019-09-13,6,125.00,250.00,375.00,500.00,625.00,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0"
]
}
}
}
}
]
}
}
Full Response Sample of Retrieving Length of Stay Rates with maxStayLength Argument
(Abbreviated, full response includes up to 734* days of rates)
Not all agencies will define rates for 734 days, so the actual number of days returned may vary depending on agency setup.
{
"data": {
"propertyManager": {
"unitsByLastRateUpdate": [
{
"unitId": 78876,
"unitRates": {
"baseRent": {
"lengthOfStay": {
"csv": [
"2023-10-02,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-03,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-04,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-05,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-06,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-07,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-08,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-09,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-10,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
"2023-10-11,8,0,0,300.00,400.00,500.00,600.00,700.00,800.00,900.00,1000.00,1100.00,1200.00,1300.00,1400.00,1500.00,1600.00,1700.00,1800.00,1900.00,2000.00,2100.00,2200.00,2300.00,2400.00,2500.00,2600.00,2700.00,2800.00,2900.00,3000.00,3100.00,3200.00,3300.00,3400.00,3500.00,3600.00,3700.00,3800.00,3900.00,4000.00,4100.00,4200.00,4300.00,4400.00,4500.00,4600.00,4700.00,4800.00,4900.00,5000.00,5100.00,5200.00,5300.00,5400.00,5500.00,5600.00,5700.00,5800.00,5900.00,6000.00,6100.00,6200.00,6300.00,6400.00,6500.00,6600.00,6700.00,6800.00,6900.00,7000.00,7100.00,7200.00,7300.00,7400.00,7500.00,7600.00,7700.00,7800.00,7900.00,8000.00,8100.00,8200.00,8300.00,8400.00,8500.00,8600.00,8700.00,8800.00,8900.00,9000.00,9100.00,9200.00,9300.00,9400.00,9500.00,9600.00,9700.00,9800.00,9900.00,0",
]
}
}
}
}
]
}
}
}
}
Full Response Sample of Using Fragments to query for Multiple Units
{
"data": {
"unit": {
"unitId": 121792,
"fees":[],
"taxRules":[
{
"amount":6,
"isPercent":true,
"applyPer":"Night",
"name":"3000.1000",
"taxRuleId":"7216",
"isTaxable":false,
"isSecondary":false,
"taxAuthority":"Dare - Occupancy TAX",
"stayDateRange":{
"min":"2017-01-01T00:00:00Z",
"max":"2017-12-31T00:00:00Z"
},
"bookDateRange":null,
"stayLengthRange":{
"min":1,
"max":90
}
},
{
"amount":6.75,
"isPercent":true,
"applyPer":"Night",
"name":"3000.1010",
"taxRuleId":"7217",
"isTaxable":false,
"isSecondary":false,
"taxAuthority":"Dare - NC St TAX",
"stayDateRange":{
"min":"2017-01-01T00:00:00Z",
"max":"9999-12-31T00:00:00Z"
},
"bookDateRange":null,
"stayLengthRange":{
"min":1,
"max":90
}
}
]
}
}
}